SceneWidget
: 3D data viewer widget#
This module provides a widget to view data sets in 3D.
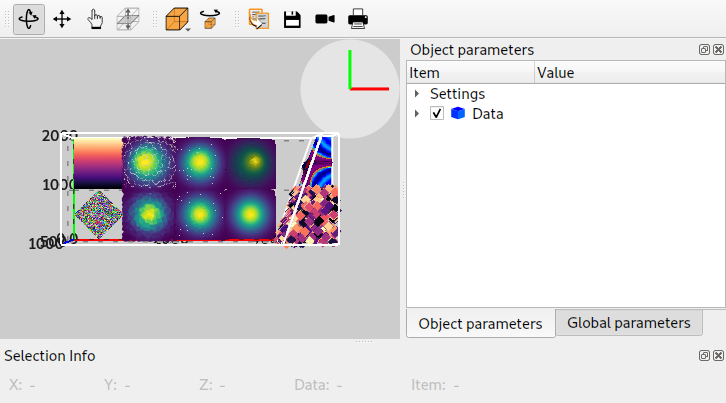
For sample code using SceneWidget
, see plot3dSceneWindow.py
in silx.gui.plot3d sample code.
SceneWidget
#
- class SceneWidget(parent=None)[source]#
Bases:
Plot3DWidget
Widget displaying data sets in 3D
- pickItems(x, y, condition=None)[source]#
Iterator over picked items in the scene at given position.
Each picked item yield a
PickingResult
object holding the picking information.It traverses the scene tree in a left-to-right top-down way.
- Parameters:
x (int) – X widget coordinate
y (int) – Y widget coordinate
condition (callable) – Optional test called for each item checking whether to process it or not.
- setInteractiveMode(mode)[source]#
Set the interactive mode.
‘panSelectedPlane’ mode set plane panning if a plane is selected, otherwise it fall backs to ‘rotate’.
- Parameters:
mode (str) – The interactive mode: ‘rotate’, ‘pan’, ‘panSelectedPlane’ or None
- addVolume(data, copy=True, index=None)[source]#
Add 3D data volume of scalar or complex to
SceneWidget
content.Dataset order is zyx (i.e., first dimension is z).
- Parameters:
data (numpy.ndarray[Union[numpy.complex64,numpy.float32]]) – 3D array of complex with shape at least (2, 2, 2)
copy (bool) – True (default) to make a copy, False to avoid copy (DO NOT MODIFY data afterwards)
index (int) – The index at which to place the item. By default it is appended to the end of the list.
- Returns:
The newly created 3D volume item
- Return type:
Union[ScalarField3D,ComplexField3D]
- add3DScatter(x, y, z, value, copy=True, index=None)[source]#
Add 3D scatter data to
SceneWidget
content.- Parameters:
x (numpy.ndarray) – Array of X coordinates (single value not accepted)
y – Points Y coordinate (array-like or single value)
z – Points Z coordinate (array-like or single value)
value – Points values (array-like or single value)
copy (bool) – True (default) to copy the data, False to use provided data (do not modify!)
index (int) – The index at which to place the item. By default it is appended to the end of the list.
- Returns:
The newly created 3D scatter item
- Return type:
- add2DScatter(x, y, value, copy=True, index=None)[source]#
Add 2D scatter data to
SceneWidget
content.Provided arrays must have the same length.
- Parameters:
x (numpy.ndarray) – X coordinates (array-like)
y (numpy.ndarray) – Y coordinates (array-like)
value – Points value: array-like or single scalar
copy (bool) – True (default) to copy the data, False to use as is (do not modify!).
index (int) – The index at which to place the item. By default it is appended to the end of the list.
- Returns:
The newly created 2D scatter item
- Return type:
- addImage(data, copy=True, index=None)[source]#
Add a 2D data or RGB(A) image to
SceneWidget
content.2D data is casted to float32. RGBA supported formats are: float32 in [0, 1] and uint8.
- Parameters:
data (numpy.ndarray) – Image as a 2D data array or RGBA image as a 3D array (height, width, channels)
copy (bool) – True (default) to copy the data, False to use as is (do not modify!).
index (int) – The index at which to place the item. By default it is appended to the end of the list.
- Returns:
The newly created image item
- Return type:
- Raises:
ValueError – For arrays of unsupported dimensions
- addItem(item, index=None)[source]#
Add an item to
SceneWidget
content- Parameters:
item (Item3D) – The item to add
index (int) – The index at which to place the item. By default it is appended to the end of the list.
- Raises:
ValueError – If the item is already in the
SceneWidget
.
- removeItem(item)[source]#
Remove an item from
SceneWidget
content.- Parameters:
item (Item3D) – The item to remove from the scene
- Raises:
ValueError – If the item does not belong to the group
- getItems()[source]#
Returns the list of
SceneWidget
items.Only items in the top-level group are returned.
- Return type:
tuple
- clearItems()[source]#
Remove all item from
SceneWidget
.
- setTextColor(color)[source]#
Set the text color.
- Parameters:
color (QColor, str or array-like of 3 or 4 float in [0., 1.] or uint8) – RGB color: name, #RRGGBB or RGB values
- setForegroundColor(color)[source]#
Set the foreground color.
- Parameters:
color (QColor, str or array-like of 3 or 4 float in [0., 1.] or uint8) – RGB color: name, #RRGGBB or RGB values
SceneWidget
items#
PickingResult
#
- class PickingResult(item, positions, indices=None, fetchdata=None)[source]#
Class to access picking information in a 3D scene.
- getData(copy=True)[source]#
Returns picked data values
- Parameters:
copy (bool) – True (default) to get a copy, False to return internal arrays
- Return type:
Union[None,numpy.ndarray]
- getPositions(frame='scene', copy=True)[source]#
Returns picking positions in item coordinates.
- Parameters:
frame (str) – The frame in which the positions are returned Either ‘scene’ for world space, ‘ndc’ for normalized device coordinates or ‘object’ for item frame.
copy (bool) – True (default) to get a copy, False to return internal arrays
- Returns:
Nx3 array of (x, y, z) coordinates
- Return type:
numpy.ndarray