profile
: Profile tool for PlotWidget#
This module provides tools to get profiles on plot data.
It provides:
ScatterProfileToolBar
: a QToolBar to handle profile on scatter data
The profile package is divided into several sub-modules.
manager
module#
This module provides a manager to compute and display profiles.
ProfileManager
class#
- class ProfileManager(parent=None, plot=None, roiManager=None)[source]#
Bases:
QObject
Base class for profile management tools
- Parameters:
plot –
PlotWidget
on which to operate.plot –
RegionOfInterestManager
on which to operate.
- setSingleProfile(enable)[source]#
Enable or disable the single profile mode.
In single mode, the manager enforce a single ROI at the same time. A new one will remove the previous one.
If this mode is not enabled, many ROIs can be created, and many profile windows will be displayed.
- isSingleProfile()[source]#
Returns true if the manager is in a single profile mode.
- Return type:
bool
- createProfileAction(profileRoiClass, parent=None)[source]#
Create an action from a class of ProfileRoi
- Parameters:
profileRoiClass (core.ProfileRoiMixIn) – A class of a profile ROI
parent (qt.QObject) – The parent of the created action.
- Return type:
qt.QAction
- createClearAction(parent)[source]#
Create an action to clean up the plot from the profile ROIs.
- Parameters:
parent (qt.QObject) – The parent of the created action.
- Return type:
qt.QAction
- createImageActions(parent)[source]#
Create actions designed for image items. This actions created new ROIs.
- Parameters:
parent (qt.QObject) – The parent of the created action.
- Return type:
List[qt.QAction]
- createScatterActions(parent)[source]#
Create actions designed for scatter items. This actions created new ROIs.
- Parameters:
parent (qt.QObject) – The parent of the created action.
- Return type:
List[qt.QAction]
- createScatterSliceActions(parent)[source]#
Create actions designed for regular scatter items. This actions created new ROIs.
This ROIs was designed to use the input data without interpolation, like you could do with an image.
- Parameters:
parent (qt.QObject) – The parent of the created action.
- Return type:
List[qt.QAction]
- createImageStackActions(parent)[source]#
Create actions designed for stack image items. This actions created new ROIs.
This ROIs was designed to create both profile on the displayed image and profile on the full stack (2D result).
- Parameters:
parent (qt.QObject) – The parent of the created action.
- Return type:
List[qt.QAction]
- createEditorAction(parent)[source]#
Create an action containing GUI to edit the selected profile ROI.
- Parameters:
parent (qt.QObject) – The parent of the created action.
- Return type:
qt.QAction
- setItemType(image=False, scatter=False)[source]#
Set the item type to use and select the active one.
- Parameters:
image (bool) – Image item are allowed
scatter (bool) – Scatter item are allowed
- setProfileWindowClass(profileWindowClass)[source]#
Set the class which will be instantiated to display profile result.
- setActiveItemTracking(tracking)[source]#
Enable/disable the tracking of the active item of the plot.
- Parameters:
tracking (bool) – Tracking mode
- setDefaultColorFromCursorColor(enabled)[source]#
Enabled/disable the use of the colormap cursor color to display the ROIs.
If set, the manager will update the color of the profile ROIs using the current colormap cursor color from the selected item.
- hasPendingOperations()[source]#
Returns true if a thread is still computing or displaying a profile.
- Return type:
bool
- requestUpdateProfile(profileRoi)[source]#
Request to update a specific profile ROI.
- Parameters:
profileRoi (ProfileRoiMixIn)
- setPlotItem(item)[source]#
Set the plot item focused by the profile manager.
- Parameters:
item (Item) – A plot item
- getDefaultColor(item)[source]#
Returns the default ROI color to use according to the given item.
- Parameters:
item (Item) – AN item
- Return type:
qt.QColor
- createProfileWindow(plot, roi)[source]#
Create a new profile window.
- Parameters:
roi (ProfileRoiMixIn) – The plot containing the raw data
roi – A managed ROI
- Return type:
~ProfileWindow
ProfileWindow
class#
- class ProfileWindow(parent=None, backend=None)[source]#
Bases:
QMainWindow
Display a computed profile.
The content can be described using
setRoiProfile()
if the source of the profile is a profile ROI, andsetProfile()
for the data content.- sigClose#
Emitted by
closeEvent()
(e.g. when the window is closed through the window manager’s close icon).
- prepareWidget(roi)[source]#
Called before the show to prepare the window to use with a specific ROI.
- createPlot1D(parent, backend)[source]#
Inherit this function to create your own plot to render 1D profiles. The default value is a Plot1D.
- Parameters:
parent – The parent of this widget or None.
backend – The backend to use for the plot. See
PlotWidget
for the list of supported backend.
- Return type:
- createPlot2D(parent, backend)[source]#
Inherit this function to create your own plot to render 2D profiles. The default value is a Plot2D.
- Parameters:
parent – The parent of this widget or None.
backend – The backend to use for the plot. See
PlotWidget
for the list of supported backend.
- Return type:
- getPlot1D(init=True)[source]#
Return the current plot used to display curves and create it if it does not yet exists and init is True. Else returns None.
- getPlot2D(init=True)[source]#
Return the current plot used to display image and create it if it does not yet exists and init is True. Else returns None.
- setRoiProfile(roi)[source]#
Set the profile ROI which it the source of the following data to display.
- Parameters:
roi (ProfileRoiMixIn) – The profile ROI data source
editors
module#
This module provides editors which are used to custom profile ROI properties.
ProfileRoiEditorAction
class#
- class ProfileRoiEditorAction(parent=None)[source]#
Bases:
QWidgetAction
Action displaying GUI to edit the selected ROI.
- Parameters:
parent (qt.QWidget) – Parent widget
- setRoiManager(roiManager)[source]#
Connect this action to a ROI manager.
- Parameters:
roiManager (RegionOfInterestManager) – A ROI manager
- setProfileRoi(roi)[source]#
Set a profile ROI to edit.
- Parameters:
roi (ProfileRoiMixIn) – A profile ROI
core
module#
This module define core objects for profile tools.
ProfileRoiMixIn
class#
- class ProfileRoiMixIn(parent=None)[source]#
Bases:
object
Base mix-in for ROI which can be used to select a profile.
This mix-in have to be applied to a
RegionOfInterest
in order to be usable by aProfileManager
.- ITEM_KIND = None#
Define the plot item which can be used with this profile ROI
- sigProfilePropertyChanged#
Emitted when a property of this profile have changed
- sigPlotItemChanged#
Emitted when the plot item linked to this profile have changed
- invalidateProfile()[source]#
Must be called by the implementation when the profile have to be recomputed.
- getPlotItem()[source]#
Returns the plot item used by this profile
- Return type:
~silx.gui.plot.items.Item
- setProfileWindow(profileWindow)[source]#
Associate a window to this ROI. Can be None.
- Parameters:
profileWindow (ProfileWindow) – A main window to display the profile.
- computeProfile(item)[source]#
Compute the profile which will be displayed.
This method is not called from the main Qt thread, but from a thread pool.
- Parameters:
item (
Item
) – A plot item- Return type:
Union
[CurveProfileData
,ImageProfileData
,RgbaProfileData
,CurvesProfileData
]
CurveProfileData
class#
ImageProfileData
class#
ScatterProfileToolBar
module#
This module profile tools for scatter plots.
ScatterProfileToolBar
#
- class ScatterProfileToolBar(parent=None, plot=None)[source]#
Bases:
ProfileToolBar
QToolBar providing scatter plot profiling tools
- Parameters:
parent – See
QToolBar
.plot –
PlotWidget
on which to operate.
rois
module#
This module define ROIs for profile tools.
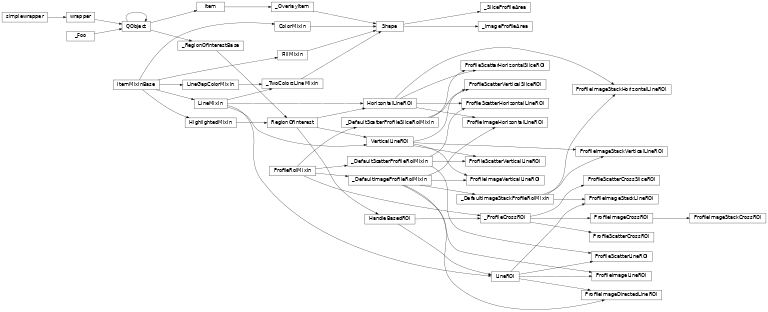