MX Calibrate#
Calibrate a translation table from a set of powder diffraction images taken at various sample-detector distances.
This is a notebook replacement of the MX-calibrate
tool from pyFAI with advanced features.
Start with some constant definition:#
[1]:
calibrant_name = "CeO2"
detector_name = "Pilatus 2M"
file_pattern = "massif1/test-powder*.cbf"
result_file = "MX-calibrate.json"
wavelength = None # set a value to override the one in the headers
[2]:
!wget http://www.silx.org/pub/pyFAI/massif1.tar.bz2
!tar -xvjf massif1.tar.bz2
--2025-03-13 15:29:54-- http://www.silx.org/pub/pyFAI/massif1.tar.bz2
Résolution de islay (islay)… 192.168.4.1
Connexion à islay (islay)|192.168.4.1|:3128… connecté.
200 OKe Proxy transmise, en attente de la réponse…
Taille : 6784503 (6,5M) [application/x-bzip2]
Sauvegarde en : « massif1.tar.bz2.5 »
massif1.tar.bz2.5 100%[===================>] 6,47M 40,4MB/s ds 0,2s
2025-03-13 15:29:54 (40,4 MB/s) — « massif1.tar.bz2.5 » sauvegardé [6784503/6784503]
massif1/
massif1/test-powder_5_0001.poni
massif1/test-powder_4_0001.cbf
massif1/test-powder_6_0001.poni
massif1/test-powder_8_0001.cbf
massif1/test-powder_7_0001.cbf
massif1/test-powder_7_0001.poni
massif1/test-powder_3_0001.cbf
massif1/test-powder_1_0001.poni
massif1/test-powder_4_0001.poni
massif1/test-powder_8_0001.poni
massif1/test-powder_2_0001.poni
massif1/test-powder_6_0001.cbf
massif1/test-powder_2_0001.cbf
massif1/test-powder_5_0001.cbf
massif1/test-powder_3_0001.poni
massif1/test-powder_1_0001.cbf
[3]:
%matplotlib widget
#inline2
[4]:
import os
import glob
import logging
import numpy
from matplotlib.pyplot import subplots
from scipy.stats import linregress
import fabio
import pyFAI
from pyFAI.gui import jupyter
import pyFAI.calibrant
from pyFAI.gui.jupyter.calib import Calibration
from pyFAI.goniometer import GeometryTransformation, GoniometerRefinement
from pyFAI.gui.cli_calibration import AbstractCalibration
import pyFAI.gui.mpl_calib
pyFAI.gui.mpl_calib.logger.setLevel(logging.ERROR)
print(f"Running pyFAI version {pyFAI.version}")
WARNING:pyFAI.gui.matplotlib:matplotlib already loaded, setting its backend may not work
Running pyFAI version 2025.3.0
[5]:
detector = pyFAI.detector_factory(detector_name)
calibrant = pyFAI.calibrant.get_calibrant(calibrant_name)
files = sorted(glob.glob(file_pattern))
print("input files: "+" ".join(files))
input files: massif1/test-powder_1_0001.cbf massif1/test-powder_2_0001.cbf massif1/test-powder_3_0001.cbf massif1/test-powder_4_0001.cbf massif1/test-powder_5_0001.cbf massif1/test-powder_6_0001.cbf massif1/test-powder_7_0001.cbf massif1/test-powder_8_0001.cbf
[6]:
first = fabio.open(files[0])
def get_dectris_headers(fimg):
"""return the dectris headers from a Pilatus detector"""
res = {}
for line in fimg.header.get("_array_data.header_contents", "").split("\n"):
words = line.split()
if len(words)>=3:
key = words[1]
for v in words[2:]:
try:
vf = float(v)
except:
continue
if not("." in v or "e" in v):
vf = int(v)
res[key] = vf
return res
get_dectris_headers(first)
[6]:
{'Silicon': 0.00045,
'Pixel_size': 0.000172,
'N_oscillations': 1,
'Chi': 0.0,
'Phi': 0.0,
'Kappa': 0.0,
'Alpha': 0.0,
'Polarization': 0.99,
'Detector_2theta': 0.0,
'Angle_increment': 1.0,
'Transmission': 100.0,
'Flux': 436215830143.2828,
'Detector_Voffset': 0.0,
'Detector_distance': 0.126474,
'Wavelength': 0.965459,
'N_excluded_pixels:': 321,
'Threshold_setting': 6421,
'Count_cutoff': 1048500,
'Tau': 0,
'Exposure_period': 0.02115,
'Exposure_time': 0.02,
'Start_angle': 0.0}
[7]:
if wavelength is None:
wavelength = get_dectris_headers(first)["Wavelength"] * 1e-10
calibrant.wavelength = wavelength
[8]:
#apply mask to the detector
mask = numpy.logical_or(detector.mask, first.data<0)
detector.mask = mask
Manual calibration of the first image#
[9]:
# Important: select the ring number before right-click on the ring. Finally click on the refine button
calib = Calibration(img=first.data,
mask=mask,
detector=detector,
wavelength=wavelength,
calibrant=calibrant)
[10]:
calib.extract_cpt()
# calib.geoRef.rot1 = calib.geoRef.rot2 = calib.geoRef.rot3 = 0
calib.refine(fixed=["wavelength", "rot3"])
Before refinement, the geometry is:
Detector Pilatus 2M PixelSize= 172µm, 172µm BottomRight (3)
Wavelength= 9.654590e-11 m
SampleDetDist= 1.001389e-01 m PONI= -3.281992e-04, 3.988812e-05 m rot1=-0.000303 rot2=0.000743 rot3=0.000000 rad
DirectBeamDist= 100.139 mm Center: x=0.408, y=-1.476 pix Tilt= 0.046° tiltPlanRotation= 67.825° 𝛌= 0.965Å
Detector Pilatus 2M PixelSize= 172µm, 172µm BottomRight (3)
Wavelength= 9.654590e-11 m
SampleDetDist= 1.001389e-01 m PONI= -3.281992e-04, 3.988812e-05 m rot1=-0.000303 rot2=0.000743 rot3=0.000000 rad
DirectBeamDist= 100.139 mm Center: x=0.408, y=-1.476 pix Tilt= 0.046° tiltPlanRotation= 67.825° 𝛌= 0.965Å
Detector Pilatus 2M PixelSize= 172µm, 172µm BottomRight (3)
Wavelength= 9.654590e-11 m
SampleDetDist= 1.001389e-01 m PONI= -3.281992e-04, 3.988812e-05 m rot1=-0.000303 rot2=0.000743 rot3=0.000000 rad
DirectBeamDist= 100.139 mm Center: x=0.408, y=-1.476 pix Tilt= 0.046° tiltPlanRotation= 67.825° 𝛌= 0.965Å
[11]:
#Return to `inline` mode
%matplotlib inline
calib.peakPicker.widget.fig.show()
Check that the beam-center and the distance is correct and how much they are off.
Calibration of all frames in automatic mode:#
[12]:
# Definition of the geometry translation function:
get_distance = lambda fimg: get_dectris_headers(fimg)["Detector_distance"]
geotrans = GeometryTransformation(param_names = ["dist_offset",
"poni1", "poni2", "rot1","rot2",
"dist_scale", "poni1_scale", "poni2_scale"],
dist_expr="pos * dist_scale + dist_offset",
poni1_expr="pos * poni1_scale + poni1",
poni2_expr="pos * poni2_scale + poni2",
rot1_expr="rot1",
rot2_expr="rot2",
rot3_expr="0.0")
param = {
"dist_offset": calib.geoRef.dist-get_distance(first),
"poni1": calib.geoRef.poni1,
"poni2": calib.geoRef.poni2,
"rot1": calib.geoRef.rot1,
"rot2": calib.geoRef.rot2,
"dist_scale": 1.0,
"poni1_scale": 0.0,
"poni2_scale": 0.0,
}
print(param)
{'dist_offset': np.float64(-0.026335086974243513), 'poni1': np.float64(-0.00032819917724852965), 'poni2': np.float64(3.9888123405063114e-05), 'rot1': np.float64(-0.00030269182833240034), 'rot2': np.float64(0.0007426536455697338), 'dist_scale': 1.0, 'poni1_scale': 0.0, 'poni2_scale': 0.0}
[13]:
# Definition of the geometry refinement: the parameter order is the same as the param_names
gonioref = GoniometerRefinement(param, #initial guess
pos_function=get_distance,
trans_function=geotrans,
detector=detector,
wavelength=wavelength)
print("Empty refinement object:")
print(gonioref)
Empty refinement object:
GoniometerRefinement with 0 geometries labeled: .
[14]:
# Let's populate the goniometer refinement object with all geometries:
for fn in files:
base = os.path.splitext(fn)[0]
fimg = fabio.open(fn)
local_calib = AbstractCalibration(img=fimg.data, mask=mask,
detector=detector,
wavelength=wavelength,
calibrant=calibrant)
local_calib.preprocess()
local_calib.fixed = ["wavelength", "rot3"]
local_calib.ai = gonioref.get_ai(get_distance(fimg))
local_calib.extract_cpt()
sg = gonioref.new_geometry(os.path.basename(base), image=fimg.data, metadata=fimg,
control_points=local_calib.peakPicker.points,
geometry=local_calib.ai,
calibrant=calibrant)
print("Filled refinement object:")
print(gonioref)
print(os.linesep+"\tLabel \t Distance")
for k, v in gonioref.single_geometries.items():
print(k,v.get_position())
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
ERROR:root:No diffraction image available => not showing the contour
Filled refinement object:
GoniometerRefinement with 8 geometries labeled: test-powder_1_0001, test-powder_2_0001, test-powder_3_0001, test-powder_4_0001, test-powder_5_0001, test-powder_6_0001, test-powder_7_0001, test-powder_8_0001.
Label Distance
test-powder_1_0001 0.126474
test-powder_2_0001 0.141749
test-powder_3_0001 0.199249
test-powder_4_0001 0.171074
test-powder_5_0001 0.226674
test-powder_6_0001 0.293162
test-powder_7_0001 0.357899
test-powder_8_0001 0.484611
[15]:
fig, ax = subplots(len(files), figsize=(10, 10*len(files)))
for sp, sg in zip(ax, gonioref.single_geometries.values()):
jupyter.display(sg=sg, ax=sp, label=sg.label)


[16]:
gonioref.refine3(fix=["dist_scale", "poni1_scale", "poni2_scale"])
Free parameters: ['dist_offset', 'poni1', 'poni2', 'rot1', 'rot2']
Fixed: {'dist_scale': 1.0, 'poni1_scale': 0.0, 'poni2_scale': 0.0}
message: Optimization terminated successfully
success: True
status: 0
fun: 0.00013456193648577567
x: [-2.720e-02 -3.975e-04 3.308e-04 -1.694e-03 3.372e-03]
nit: 9
jac: [ 2.071e-07 1.828e-07 1.802e-07 -4.625e-08 5.059e-08]
nfev: 58
njev: 9
Constrained Least square 0.0001358990033245626 --> 0.00013456193648577567
maxdelta on rot2: 0.0007426536455697338 --> 0.003372023836199041
[16]:
np.float64(0.00013456193648577567)
[17]:
gonioref.refine3(fix=[])
Free parameters: ['dist_offset', 'poni1', 'poni2', 'rot1', 'rot2', 'dist_scale', 'poni1_scale', 'poni2_scale']
Fixed: {}
message: Optimization terminated successfully
success: True
status: 0
fun: 0.00013384162837038897
x: [-2.506e-02 -1.532e-03 -8.249e-04 6.814e-04 2.154e-03
9.898e-01 7.022e-03 8.464e-03]
nit: 30
jac: [-1.275e-08 -6.858e-10 9.670e-09 -3.241e-09 -1.104e-08
-6.026e-09 7.116e-09 -8.198e-09]
nfev: 272
njev: 30
Constrained Least square 0.00013456193648577567 --> 0.00013384162837038897
maxdelta on dist_scale: 1.0 --> 0.9897531676124213
[17]:
np.float64(0.00013384162837038897)
Interpretation of this fit:
[18]:
gonioref.get_ai(0.2)
[18]:
Detector Pilatus 2M PixelSize= 172µm, 172µm BottomRight (3)
Wavelength= 9.654590e-11 m
SampleDetDist= 1.728884e-01 m PONI= -1.277825e-04, 8.678612e-04 m rot1=0.000681 rot2=0.002154 rot3=0.000000 rad
DirectBeamDist= 172.889 mm Center: x=4.361, y=1.422 pix Tilt= 0.129° tiltPlanRotation= 107.555° 𝛌= 0.965Å
[19]:
gonioref.get_ai(0.3)
[19]:
Detector Pilatus 2M PixelSize= 172µm, 172µm BottomRight (3)
Wavelength= 9.654590e-11 m
SampleDetDist= 2.718638e-01 m PONI= 5.744527e-04, 1.714245e-03 m rot1=0.000681 rot2=0.002154 rot3=0.000000 rad
DirectBeamDist= 271.864 mm Center: x=8.890, y=6.744 pix Tilt= 0.129° tiltPlanRotation= 107.555° 𝛌= 0.965Å
Persistence of this fit
[20]:
gonioref.save(result_file)
[21]:
with open(result_file) as r:
print(r.read())
{
"content": "Goniometer calibration v2",
"detector": "Pilatus 2M",
"detector_config": {
"orientation": 3
},
"wavelength": 9.65459e-11,
"param": [
-0.02506219908392629,
-0.0015322527085403072,
-0.0008249070438579754,
0.0006813851321719287,
0.0021538371006793585,
0.9897531676124213,
0.007022351289970616,
0.00846384112636606
],
"param_names": [
"dist_offset",
"poni1",
"poni2",
"rot1",
"rot2",
"dist_scale",
"poni1_scale",
"poni2_scale"
],
"pos_names": [
"pos"
],
"trans_function": {
"content": "GeometryTransformation",
"param_names": [
"dist_offset",
"poni1",
"poni2",
"rot1",
"rot2",
"dist_scale",
"poni1_scale",
"poni2_scale"
],
"pos_names": [
"pos"
],
"dist_expr": "pos * dist_scale + dist_offset",
"poni1_expr": "pos * poni1_scale + poni1",
"poni2_expr": "pos * poni2_scale + poni2",
"rot1_expr": "rot1",
"rot2_expr": "rot2",
"rot3_expr": "0.0",
"constants": {
"pi": 3.141592653589793
}
}
}
Interpretation of the fit:
[22]:
distances = []
f_distances = []
f_poni1 = []
f_poni2 = []
g_distances = []
g_poni1 = []
g_poni2 = []
for sg in gonioref.single_geometries.values():
distance = sg.get_position()
distances.append(distance)
sg.geometry_refinement.refine3(fix=["wavelength", "rot3"])
f_distances.append(sg.geometry_refinement.dist)
f_poni1.append(sg.geometry_refinement.poni1)
f_poni2.append(sg.geometry_refinement.poni2)
ai = gonioref.get_ai(distance)
g_distances.append(ai.dist)
g_poni1.append(ai.poni1)
g_poni2.append(ai.poni2)
order = numpy.argsort(distances)
distances = numpy.take(distances, order)
f_distances = numpy.take(f_distances, order)
f_poni1 = numpy.take(f_poni1, order)
f_poni2 = numpy.take(f_poni2, order)
g_distances = numpy.take(g_distances, order)
g_poni1 = numpy.take(g_poni1, order)
g_poni1 = numpy.take(g_poni1, order)
[23]:
fig,ax = subplots(2, figsize=(8,10))
ax[0].plot(distances, f_distances, "1", label="fitted individually")
ax[0].plot(distances, g_distances, label="fitted table")
ax[0].set_title("Observed deviations:")
ax[1].set_xlabel("Encoder distance (m)")
ax[1].plot(distances, f_poni1, "1", label="poni1 fitted individually")
ax[1].plot(distances, g_poni1, label="poni1 fitted table")
ax[1].plot(distances, f_poni2, "1", label="poni2 fitted individually")
ax[1].plot(distances, g_poni2, label="poni2 fitted table")
ax[0].set_ylabel("Fitted distance (m)")
ax[1].set_ylabel("Fitted PONIs (m)")
ax[0].legend()
ax[1].legend()
pass
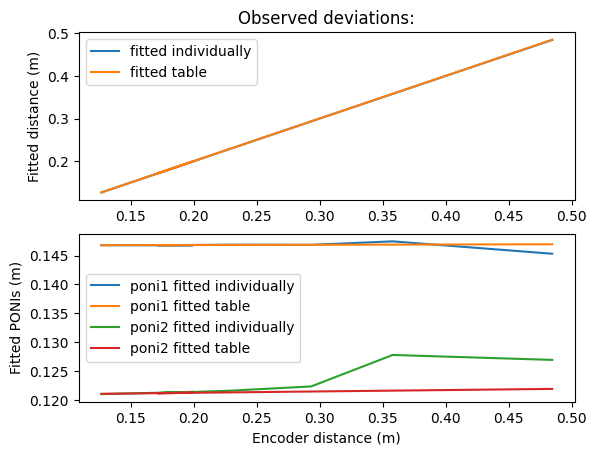
[24]:
obtained_x = []
obtained_y = []
for dst in distances:
geo = gonioref.get_ai(dst)
fit2d = geo.getFit2D()
obtained_x.append(fit2d["centerX"])
obtained_y.append(fit2d["centerY"])
fig,ax = subplots()
ax.plot(distances, obtained_x, label="X")
ax.plot(distances, obtained_y, label="Y")
ax.set_title("Observed variation:")
ax.set_ylabel("Beam center (pixels)")
ax.set_xlabel("Encoder distance (m)")
ax.legend()
pass
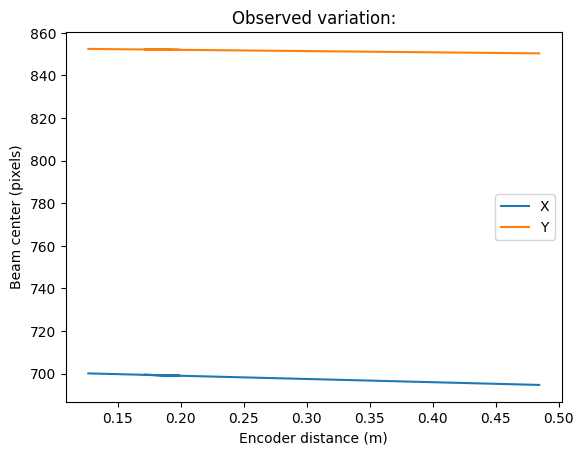
[25]:
#Simply print out the result:
lrx = linregress(distances, obtained_x)
lry = linregress(distances, obtained_y)
print(f"Beam-center X: {lrx}")
print(f"Beam-center y: {lry}")
print()
print(f"beamcenter_x = {lrx.intercept:.3f} {lrx.slope:+.3f} * encoder_value")
print(f"beamcenter_y = {lry.intercept:.3f} {lry.slope:+.3f} * encoder_value")
Beam-center X: LinregressResult(slope=np.float64(45.287429820133504), intercept=np.float64(-4.696686228952235), rvalue=np.float64(1.0), pvalue=np.float64(2.5000000000000343e-60), stderr=np.float64(0.0), intercept_stderr=np.float64(0.0))
Beam-center y: LinregressResult(slope=np.float64(53.221640547693454), intercept=np.float64(-9.222283132292265), rvalue=np.float64(1.0), pvalue=np.float64(2.5000000000000343e-60), stderr=np.float64(0.0), intercept_stderr=np.float64(0.0))
beamcenter_x = -4.697 +45.287 * encoder_value
beamcenter_y = -9.222 +53.222 * encoder_value
Nota:
The degradation between 0.3 and 0.5m correspond to the image 6->7 and is related to the disparition of the third ring!
Conclusion:#
This notebook demonstrates:
The usage of the geometry calibration in Jupyter-lab to calibrate the first image
The creation of a goniometer-refinement
The population of this goniometer-refinement with automatic control-point extraction
The fit of the table, first with the constrains of a perfecty aligned table, then with a mis-aligned table
In our case the table is miss-aligned in the horizontal direction by 2.3mm/meter (i.e. 2.3 mradian). This should be taken into account when calculating the beam-center at different distances.