tools
: Tool widgets for PlotWidget¶
This package provides a set of widgets working with PlotWidget
.
It provides some QToolBar and QWidget:
It also provides a roi
module to handle
interactive region of interest on a PlotWidget
.
PositionInfo
class¶
-
class
PositionInfo
(parent=None, plot=None, converters=None)[source]¶ Bases:
PySide2.QtWidgets.QWidget
QWidget displaying coords converted from data coords of the mouse.
Provide this widget with a list of couple:
- A name to display before the data
- A function that takes (x, y) as arguments and returns something that gets converted to a string. If the result is a float it is converted with ‘%.7g’ format.
To run the following sample code, a QApplication must be initialized. First, create a PlotWindow and add a QToolBar where to place the PositionInfo widget.
>>> from silx.gui.plot import PlotWindow >>> from silx.gui import qt
>>> plot = PlotWindow() # Create a PlotWindow to add the widget to >>> toolBar = qt.QToolBar() # Create a toolbar to place the widget in >>> plot.addToolBar(qt.Qt.BottomToolBarArea, toolBar) # Add it to plot
Then, create the PositionInfo widget and add it to the toolbar. The PositionInfo widget is created with a list of converters, here to display polar coordinates of the mouse position.
>>> import numpy >>> from silx.gui.plot.tools import PositionInfo
>>> position = PositionInfo(plot=plot, converters=[ ... ('Radius', lambda x, y: numpy.sqrt(x*x + y*y)), ... ('Angle', lambda x, y: numpy.degrees(numpy.arctan2(y, x)))]) >>> toolBar.addWidget(position) # Add the widget to the toolbar <...> >>> plot.show() # To display the PlotWindow with the position widget
Parameters: - plot – The PlotWidget this widget is displaying data coords from.
- converters – List of 2-tuple: name to display and conversion function from (x, y) in data coords to displayed value. If None, the default, it displays X and Y.
- parent – Parent widget
-
getPlotWidget
()[source]¶ Returns the PlotWidget this widget is attached to or None.
Return type: Union[PlotWidget,None]
-
SNAPPING_DISABLED
= 0¶ No snapping occurs
-
SNAPPING_CROSSHAIR
= 1¶ Snapping only enabled when crosshair cursor is enabled
-
SNAPPING_ACTIVE_ONLY
= 2¶ Snapping only enabled for active item
-
SNAPPING_SYMBOLS_ONLY
= 4¶ Snapping only when symbols are visible
-
SNAPPING_CURVE
= 8¶ Snapping on curves
-
SNAPPING_SCATTER
= 16¶ Snapping on scatter
LimitsToolBar
class¶
-
class
LimitsToolBar
(parent=None, plot=None, title='Limits')[source]¶ Bases:
PySide2.QtWidgets.QToolBar
QToolBar displaying and controlling the limits of a
PlotWidget
.To run the following sample code, a QApplication must be initialized. First, create a PlotWindow:
>>> from silx.gui.plot import PlotWindow >>> plot = PlotWindow() # Create a PlotWindow to add the toolbar to
Then, create the LimitsToolBar and add it to the PlotWindow.
>>> from silx.gui import qt >>> from silx.gui.plot.tools import LimitsToolBar
>>> toolbar = LimitsToolBar(plot=plot) # Create the toolbar >>> plot.addToolBar(qt.Qt.BottomToolBarArea, toolbar) # Add it to the plot >>> plot.show() # To display the PlotWindow with the limits toolbar
Parameters: - parent – See
QToolBar
. - plot –
PlotWidget
instance on which to operate. - title (str) – See
QToolBar
.
-
plot
¶ The
PlotWidget
the toolbar is attached to.
- parent – See
InteractiveModeToolBar
class¶
-
class
InteractiveModeToolBar
(parent=None, plot=None, title='Plot Interaction')[source]¶ Bases:
PySide2.QtWidgets.QToolBar
Toolbar with interactive mode actions
Parameters: - parent – See
QWidget
- plot (silx.gui.plot.PlotWidget) – PlotWidget to control
- title (str) – Title of the toolbar.
-
getZoomModeAction
()[source]¶ Returns the zoom mode QAction.
Return type: PlotAction
-
getPanModeAction
()[source]¶ Returns the pan mode QAction
Return type: PlotAction
- parent – See
OutputToolBar
class¶
-
class
OutputToolBar
(parent=None, plot=None, title='Plot Output')[source]¶ Bases:
PySide2.QtWidgets.QToolBar
Toolbar providing icons to copy, save and print a PlotWidget
Parameters: - parent – See
QWidget
- plot (silx.gui.plot.PlotWidget) – PlotWidget to control
- title (str) – Title of the toolbar.
-
getCopyAction
()[source]¶ Returns the QAction performing copy to clipboard of the PlotWidget
Return type: PlotAction
-
getSaveAction
()[source]¶ Returns the QAction performing save to file of the PlotWidget
Return type: PlotAction
-
getPrintAction
()[source]¶ Returns the QAction performing printing of the PlotWidget
Return type: PlotAction
- parent – See
ImageToolBar
class¶
-
class
ImageToolBar
(parent=None, plot=None, title='Image')[source]¶ Bases:
PySide2.QtWidgets.QToolBar
Toolbar providing PlotAction suited when displaying images
Parameters: - parent – See
QWidget
- plot (silx.gui.plot.PlotWidget) – PlotWidget to control
- title (str) – Title of the toolbar.
-
getResetZoomAction
()[source]¶ Returns the QAction to reset the zoom.
Return type: PlotAction
-
getColormapAction
()[source]¶ Returns the QAction to control the colormap.
Return type: PlotAction
- parent – See
CurveToolBar
class¶
-
class
CurveToolBar
(parent=None, plot=None, title='Image')[source]¶ Bases:
PySide2.QtWidgets.QToolBar
Toolbar providing PlotAction suited when displaying curves
Parameters: - parent – See
QWidget
- plot (silx.gui.plot.PlotWidget) – PlotWidget to control
- title (str) – Title of the toolbar.
-
getResetZoomAction
()[source]¶ Returns the QAction to reset the zoom.
Return type: PlotAction
-
getXAxisAutoScaleAction
()[source]¶ Returns the QAction to toggle X axis autoscale.
Return type: PlotAction
-
getYAxisAutoScaleAction
()[source]¶ Returns the QAction to toggle Y axis autoscale.
Return type: PlotAction
-
getXAxisLogarithmicAction
()[source]¶ Returns the QAction to toggle X axis log/linear scale.
Return type: PlotAction
-
getYAxisLogarithmicAction
()[source]¶ Returns the QAction to toggle Y axis log/linear scale.
Return type: PlotAction
-
getGridAction
()[source]¶ Returns the action to toggle the plot grid.
Return type: PlotAction
-
getCurveStyleAction
()[source]¶ Returns the QAction to change the style of all curves.
Return type: PlotAction
- parent – See
ScatterToolBar
class¶
-
class
ScatterToolBar
(parent=None, plot=None, title='Scatter Tools')[source]¶ Bases:
PySide2.QtWidgets.QToolBar
Toolbar providing PlotAction suited when displaying scatter plot
Parameters: - parent – See
QWidget
- plot (silx.gui.plot.PlotWidget) – PlotWidget to control
- title (str) – Title of the toolbar.
-
getResetZoomAction
()[source]¶ Returns the QAction to reset the zoom.
Return type: PlotAction
-
getXAxisLogarithmicAction
()[source]¶ Returns the QAction to toggle X axis log/linear scale.
Return type: PlotAction
-
getYAxisLogarithmicAction
()[source]¶ Returns the QAction to toggle Y axis log/linear scale.
Return type: PlotAction
-
getGridAction
()[source]¶ Returns the action to toggle the plot grid.
Return type: PlotAction
-
getColormapAction
()[source]¶ Returns the QAction to control the colormap.
Return type: PlotAction
-
getSymbolToolButton
()[source]¶ Returns the QToolButton controlling symbol size and marker.
Return type: SymbolToolButton
- parent – See
CurveLegendsWidget
¶
This module provides a widget to display PlotWidget
curve legends.
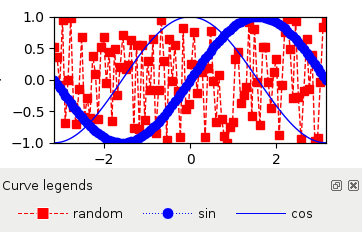
-
class
CurveLegendsWidget
(parent=None)[source]¶ Bases:
PySide2.QtWidgets.QWidget
Widget displaying curves legends in a plot
Parameters: parent (QWidget) – See QWidget
-
sigCurveClicked
= <PySide2.QtCore.Signal object>¶ Signal emitted when the legend of a curve is clicked
It provides the corresponding curve.
-
roi
: Region of interest¶
This module provides ROI interaction for PlotWidget
.
RegionOfInterestManager
class¶
-
class
RegionOfInterestManager
(parent)[source]¶ Class handling ROI interaction on a PlotWidget.
It supports the multiple ROIs: points, rectangles, polygons, lines, horizontal and vertical lines.
See
plotInteractiveImageROI.py
sample code (Sample Code).Parameters: parent (silx.gui.plot.PlotWidget) – The plot widget in which to control the ROIs. -
sigRoiAdded
= <PySide2.QtCore.Signal object>¶ Signal emitted when a new ROI has been added.
It provides the newly add
RegionOfInterest
object.
-
sigRoiAboutToBeRemoved
= <PySide2.QtCore.Signal object>¶ Signal emitted just before a ROI is removed.
It provides the
RegionOfInterest
object that is about to be removed.
-
sigRoiChanged
= <PySide2.QtCore.Signal object>¶ Signal emitted whenever the ROIs have changed.
-
sigInteractiveModeStarted
= <PySide2.QtCore.Signal object>¶ Signal emitted when switching to ROI drawing interactive mode.
It provides the class of the ROI which will be created by the interactive mode.
-
sigInteractiveModeFinished
= <PySide2.QtCore.Signal object>¶ Signal emitted when leaving and interactive ROI drawing.
It provides the list of ROIs.
-
classmethod
getSupportedRoiClasses
()[source]¶ Returns the default available ROI classes
Return type: List[class]
-
getInteractionModeAction
(roiClass)[source]¶ Returns the QAction corresponding to a kind of ROI
The QAction allows to enable the corresponding drawing interactive mode.
Parameters: roiClass (str) – The ROI class which will be crated by this action. Return type: QAction Raises: ValueError – If kind is not supported
-
getRois
()[source]¶ Returns the list of ROIs.
It returns an empty tuple if there is currently no ROI.
Returns: Tuple of arrays of objects describing the ROIs Return type: List[RegionOfInterest]
-
createRoi
(roiClass, points, label='', index=None)[source]¶ Create a new ROI and add it to list of ROIs.
Parameters: - roiClass (class) – The class of the ROI to create
- points (numpy.ndarray) – The first shape used to create the ROI
- label (str) – The label to display along with the ROI.
- index (int) – The position where to insert the ROI. By default it is appended to the end of the list.
Returns: The created ROI object
Return type: roi_items.RegionOfInterest
Raises: RuntimeError – When ROI cannot be added because the maximum number of ROIs has been reached.
-
addRoi
(roi, index=None, useManagerColor=True)[source]¶ Add the ROI to the list of ROIs.
Parameters: - roi (roi_items.RegionOfInterest) – The ROI to add
- index (int) – The position where to insert the ROI, By default it is appended to the end of the list of ROIs
Raises: RuntimeError – When ROI cannot be added because the maximum number of ROIs has been reached.
-
removeRoi
(roi)[source]¶ Remove a ROI from the list of ROIs.
Parameters: roi (roi_items.RegionOfInterest) – The ROI to remove Raises: ValueError – When ROI does not belong to this object
-
setColor
(color)[source]¶ Set the default color to use when creating ROIs.
Existing ROIs are not affected.
Parameters: color – The color to use for displaying ROIs as either a color name, a QColor, a list of uint8 or float in [0, 1].
-
getCurrentInteractionModeRoiClass
()[source]¶ Returns the current ROI class used by the interactive drawing mode.
Returns None if the ROI manager is not in an interactive mode.
Return type: Union[class,None]
-
start
(roiClass)[source]¶ Start an interactive ROI drawing mode.
Parameters: roiClass (class) – The ROI class to create. It have to inherite from roi_items.RegionOfInterest. Returns: True if interactive ROI drawing was started, False otherwise Return type: bool Raises: ValueError – If roiClass is not supported
-
stop
()[source]¶ Stop interactive ROI drawing mode.
Returns: True if an interactive ROI drawing mode was actually stopped Return type: bool
-
InteractiveRegionOfInterestManager
class¶
-
class
InteractiveRegionOfInterestManager
(parent)[source]¶ RegionOfInterestManager with features for use from interpreter.
It is meant to be used through the
exec_()
. It provides some messages to display in a status bar and different modes to end blocking calls toexec_()
.Parameters: parent – See QObject -
sigMessageChanged
= <PySide2.QtCore.Signal object>¶ Signal emitted when a new message should be displayed to the user
It provides the message as a str.
-
getMaxRois
()[source]¶ Returns the maximum number of ROIs or None if no limit.
Return type: Union[int,None]
-
setMaxRois
(max_)[source]¶ Set the maximum number of ROIs.
Parameters: max (Union[int,None]) – The max limit or None for no limit. Raises: ValueError – If there is more ROIs than max value
-
class
ValidationMode
[source]¶ Mode of validation to leave blocking
exec_()
-
AUTO
= <ValidationMode.AUTO: 'auto'>¶ Automatically ends the interactive mode once the user terminates the last ROI shape.
-
ENTER
= <ValidationMode.ENTER: 'enter'>¶ Ends the interactive mode when the Enter key is pressed.
-
AUTO_ENTER
= <ValidationMode.AUTO_ENTER: 'auto_enter'>¶ Ends the interactive mode when reaching max ROIs or when the Enter key is pressed.
-
NONE
= <ValidationMode.NONE: 'none'>¶ Do not provide the user a way to end the interactive mode.
The end of
exec_()
is done throughquit()
or timeout.
-
-
getValidationMode
()[source]¶ Returns the interactive mode validation in use.
Return type: ValidationMode
-
setValidationMode
(mode)[source]¶ Set the way to perform interactive mode validation.
See
ValidationMode
enumeration for the supported validation modes.Parameters: mode (ValidationMode) – The interactive mode validation to use.
-
getMessage
()[source]¶ Returns the current status message.
This message is meant to be displayed in a status bar.
Return type: str
-
exec_
(roiClass, timeout=0)[source]¶ Block until ROI selection is done or timeout is elapsed.
quit()
also ends this blocking call.Parameters: - roiClass (class) – The class of the ROI which have to be created. See silx.gui.plot.items.roi.
- timeout (int) – Maximum duration in seconds to block. Default: No timeout
Returns: The list of ROIs
Return type: List[RegionOfInterest]
-
RegionOfInterestTableWidget
class¶
-
class
RegionOfInterestTableWidget
(parent=None)[source]¶ Widget displaying the ROIs of a
RegionOfInterestManager
-
setRegionOfInterestManager
(manager)[source]¶ Set the
RegionOfInterestManager
object to sync withParameters: manager (RegionOfInterestManager) –
-
getRegionOfInterestManager
()[source]¶ Returns the
RegionOfInterestManager
this widget supervise.It returns None if not sync with an
RegionOfInterestManager
.Return type: RegionOfInterestManager
-
profile
: Profile Tools¶
This module provides tools to get profiles on plot data.
It provides:
ScatterProfileToolBar
: a QToolBar to handle profile on scatter data
ScatterProfileToolBar
¶
-
class
ScatterProfileToolBar
(parent=None, plot=None, title='Scatter Profile')[source]¶ QToolBar providing scatter plot profiling tools
Parameters: - parent – See
QToolBar
. - plot –
PlotWidget
on which to operate. - title (str) – See
QToolBar
.
-
clearProfile
()¶ Clear the current line ROI and associated profile
-
getColor
()¶ Returns the color used for the profile and ROI
Return type: QColor
-
getDefaultProfileWindow
()¶ Returns the default floating profile window if in use else None.
See
isDefaultProfileWindowEnabled()
Return type: Union[ProfileMainWindow,None]
-
getPlotWidget
()¶ The
PlotWidget
associated to the toolbar.Return type: Union[PlotWidget,None]
-
getProfilePoints
(copy=True)¶ Returns the profile sampling points as (x, y) or None
Parameters: copy (bool) – True to get a copy, False to get internal arrays (do not modify) Return type: Union[numpy.ndarray,None]
-
getProfileTitle
()¶ Returns the profile title
Return type: str
-
getProfileValues
(copy=True)¶ Returns the values of the profile or None
Parameters: copy (bool) – True to get a copy, False to get internal arrays (do not modify) Return type: Union[numpy.ndarray,None]
-
isDefaultProfileWindowEnabled
()¶ Returns True if the default floating profile window is used
Return type: bool
-
setColor
(color)¶ Set the color to use for ROI and profile.
Parameters: color – Either a color name, a QColor, a list of uint8 or float in [0, 1].
-
setDefaultProfileWindowEnabled
(enabled)¶ Set whether to use or not the default floating profile window.
Parameters: enabled (bool) – True to use, False to disable
- parent – See
ColorBar
: ColorBar Widget¶
Module containing several widgets associated to a colormap.
ColorBarWidget
class¶
-
class
ColorBarWidget
(parent=None, plot=None, legend=None)[source]¶ Colorbar widget displaying a colormap
It uses a description of colormap as dict compatible with
Plot
.To run the following sample code, a QApplication must be initialized.
>>> from silx.gui.plot import Plot2D >>> from silx.gui.plot.ColorBar import ColorBarWidget
>>> plot = Plot2D() # Create a plot widget >>> plot.show()
>>> colorbar = ColorBarWidget(plot=plot, legend='Colormap') # Associate the colorbar with it >>> colorbar.show()
Initializer parameters:
Parameters: - parent – See
QWidget
- plot – PlotWidget the colorbar is attached to (optional)
- legend (str) – the label to set to the colorbar
-
sigVisibleChanged
= <PySide2.QtCore.Signal object>¶ Emitted when the property visible have changed.
-
setPlot
(plot)[source]¶ Associate a plot to the ColorBar
Parameters: plot – the plot to associate with the colorbar. If None will remove any connection with a previous plot.
-
setColormap
(colormap, data=None)[source]¶ Set the colormap to be displayed.
Parameters: - colormap (Colormap) – The colormap to apply on the ColorBarWidget
- data (numpy.ndarray) – the data to display, needed if the colormap require an autoscale
-
setLegend
(legend)[source]¶ Set the legend displayed along the colorbar
Parameters: legend (str) – The label
- parent – See