PrintPreviewToolButton
: Print preview buttons¶
This modules provides tool buttons to send the content of a plot to a print preview page. The plot content can then be moved on the page and resized prior to printing.
Examples¶
Simple example¶
from silx.gui import qt
from silx.gui.plot import PlotWidget
from silx.gui.plot.PrintPreviewToolButton import PrintPreviewToolButton
import numpy
app = qt.QApplication([])
pw = PlotWidget()
toolbar = qt.QToolBar(pw)
toolbutton = PrintPreviewToolButton(parent=toolbar, plot=pw)
pw.addToolBar(toolbar)
toolbar.addWidget(toolbutton)
pw.show()
x = numpy.arange(1000)
y = x / numpy.sin(x)
pw.addCurve(x, y)
app.exec_()
Singleton example¶
This example illustrates how to print the content of several different
plots on the same page. The plots all instantiate a
SingletonPrintPreviewToolButton
, which relies on a singleton widget
(silx.gui.widgets.PrintPreview.SingletonPrintPreviewDialog
).
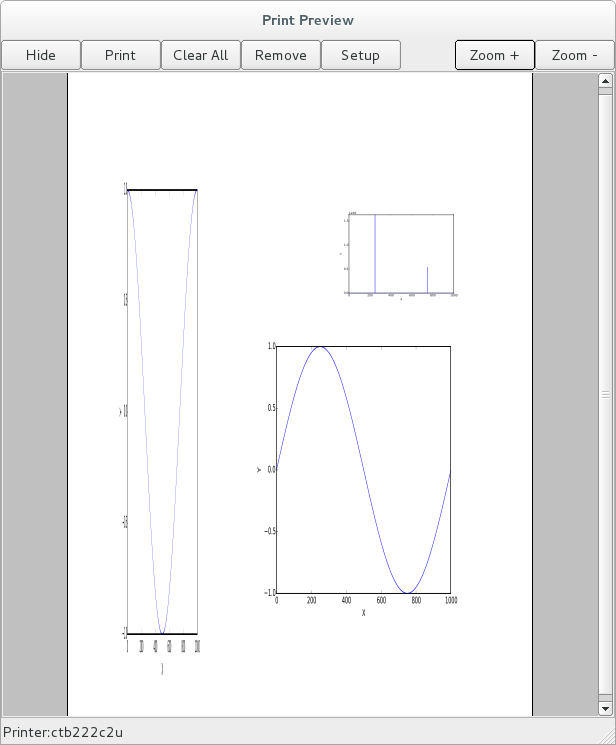
from silx.gui import qt
from silx.gui.plot import PlotWidget
from silx.gui.plot.PrintPreviewToolButton import SingletonPrintPreviewToolButton
import numpy
app = qt.QApplication([])
plot_widgets = []
for i in range(3):
pw = PlotWidget()
toolbar = qt.QToolBar(pw)
toolbutton = SingletonPrintPreviewToolButton(parent=toolbar,
plot=pw)
pw.addToolBar(toolbar)
toolbar.addWidget(toolbutton)
pw.show()
plot_widgets.append(pw)
x = numpy.arange(1000)
plot_widgets[0].addCurve(x, numpy.sin(x * 2 * numpy.pi / 1000))
plot_widgets[1].addCurve(x, numpy.cos(x * 2 * numpy.pi / 1000))
plot_widgets[2].addCurve(x, numpy.tan(x * 2 * numpy.pi / 1000))
app.exec_()
-
class
PrintPreviewToolButton
(parent=None, plot=None)[source]¶ QToolButton to open a
PrintPreviewDialog
(if not already open) and add the current plot to its page to be printed.Parameters: - parent – See
QAction
- plot –
PlotWidget
instance on which to operate
-
printPreviewDialog
¶ Lazy loaded
PrintPreviewDialog
-
getTitle
()[source]¶ Implement this method to fetch the title in the plot.
Returns: Title to be printed above the plot, or None (no title added) Return type: str or None
-
getCommentAndPosition
()[source]¶ Implement this method to fetch the legend to be printed below the figure and its position.
Returns: Legend to be printed below the figure and its position: “CENTER”, “LEFT” or “RIGHT” Return type: (str, str) or (None, None)
-
getPlot
()[source]¶ Return the
PlotWidget
associated with this tool button.Return type: PlotWidget
- parent – See
-
class
SingletonPrintPreviewToolButton
(parent=None, plot=None)[source]¶ This class is similar to its parent class
PrintPreviewToolButton
but it uses a singleton print preview widget.This allows for several plots to send their content to the same print page, and for users to arrange them.
-
printPreviewDialog
¶ Lazy loaded
PrintPreviewDialog
-